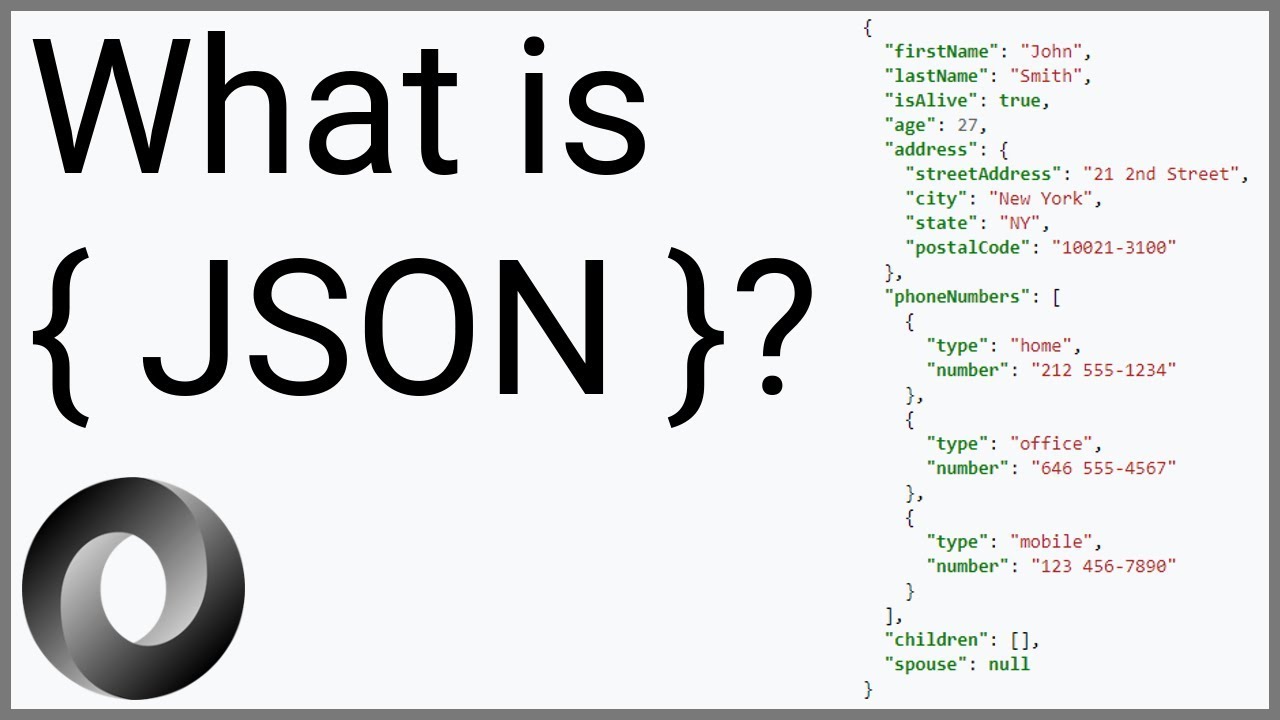
Fastjson
json string > List
1 | List<User> userList = JSON.parseArray(userStr, User.class); |
SimplePropertyPreFilter
剔除某个属性
1 | // 去掉 product 中的 productText 字段 |
自定义序列化与反序列化
自定义序列化
转换 productTitle 字段中包含的 HTML 特殊字符
自定义序列化器
1
2
3
4
5
6
7
8
9
10
11
12
13
14import org.apache.commons.text.StringEscapeUtils;
public class StringEscapeSerialization implements ObjectSerializer {
public void write(JSONSerializer serializer, Object object, Object fieldName, Type fieldType, int features) throws IOException {
SerializeWriter out = serializer.out;
if (object == null) {
out.writeNull();
} else if (object instanceof String) {
out.writeString(StringEscapeUtils.unescapeHtml4((String) object));
}
}
}使用自定义的序列化器
1
2
3
4
5public class Product {
.class) (serializeUsing= StringEscapeSerialization
private String productTitle;
}测试
1
2
3
4
5
6public static void main(String[] args) {
Product product = new Product();
product.setProductTitle("iPhone X Pro "HK"");
String s = JSON.toJSONString(product);
System.out.println(s);
}结果
1
{"productTitle":"iPhone X Pro \"HK\""}