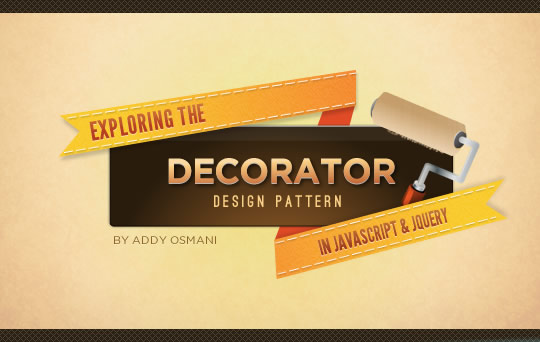
装饰者模式
别称:包装模式、装饰器模式、Wrapper、Decorator
装饰器模式(Decorator Pattern)允许在不改变其结构的情况下向一个现有的对象添加新的功能。
结构
- 抽象构件 (Component)
- 具体构件 (Concrete Component)
- 抽象装饰类 (Decorator)
- 具体装饰类 (Concrete Decorators)
简单示例
画图形:图形有长方形、圆形;可以画红色的也可以画绿色的。
抽象构件
1 | public interface Shape { |
具体构件
1 | public class Rectangle implements Shape { |
1 | public class Circle implements Shape { |
抽象装饰类
1 | public abstract class ShapeDecorator implements Shape { |
具体装饰类
1 | public class RedShapeDecorator extends ShapeDecorator { |
1 | public class GreenShapeDecorator extends ShapeDecorator { |
客户端使用
1 | public static void main(String[] args) { |
1 | Shape: rectangle Color: red |
典型应用
Java I/O
抽象构件:java.io.InputStream
具体构件:
java.io.FileInputStream
java.io.ByteArrayInputStream
java.io.PipedInputStream
抽象装饰类:java.io.FilterInputStream
具体装饰类:
java.io.BufferedInputStream
java.io.DataInputStream
java.io.PushbackInputStream
实例化一个具有缓存功能的字节流对象时,只需要在 FileInputStream 对象上再套一层 BufferedInputStream 对象即可。
1 | FileInputStream fileInputStream = new FileInputStream(filePath); |
DataInputStream 装饰者提供了对更多数据类型进行输入的操作,比如 int、double 等基本类型。